Build Your First App
To learn about the SDK's functionality, let's build a TruffleEcho app.
When asked "Who is Truffle?", your Agent will return:
"The great and mighty Truffle? All hail our overlord!"
A silly little example that will show exactly how you can get started in 5 minutes.
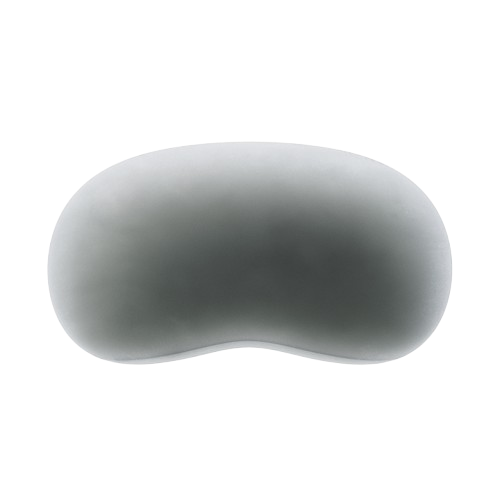
A simple app too slow for you? Jump to our more technical Deep Dive 🡒
Transform Python into Agent Tools​
The Truffle SDK allows you to write simple Python functions that get magically transformed into usable and recognizable tools to empower your Truffle Agent.
The SDK automagically integrates with your Truffle Client MacOS app, so you'll be able to use your new tools right away.
Context​
Let's talk context! We'll be using a few key terms, and it's important we're all on the same page:
Prompt: This is what you type into the Truffle MacOS client – it's how you talk to your Agent.
App: Think of this as a tool, or a bundle of tools, that you create for your Agent to use.
Truffle SDK: This is the toolkit (accessed via the CLI) that lets you build these apps.
Prerequisites​
Before you install the Truffle SDK, please ensure your environment is set up correctly. This will help avoid common issues and keep your Python projects isolated and manageable.
1. Check Python Version​
Truffle requires Python 3.10 or later. Check your Python version:
python3 --version
If you see a version lower than 3.10, or get a "command not found" error, install Python 3.10+ for your platform:
- Ubuntu/Debian:
sudo apt update
sudo apt install python3 python3-venv python3-pip - MacOS (with Homebrew):
brew install [email protected]
- Windows (with Chocolatey):
Or download from python.org.
choco install python --version=3.10.0
2. Ensure venv
is Available​
A virtual environment keeps your dependencies isolated. If python3 -m venv
fails, install venv:
- Ubuntu/Debian:
sudo apt install python3-venv
- MacOS: (venv is included with Homebrew Python)
- Windows: (venv is included by default)
3. (Recommended) Create and Activate a Virtual Environment​
python3 -m venv truffle-env
# Activate the environment:
# On Linux/MacOS:
source truffle-env/bin/activate
# On Windows:
truffle-env\Scripts\activate
Virtual environments prevent dependency conflicts and make it easy to manage your project's packages. Always activate your environment before installing or running Truffle SDK commands.
Set Up Your Environment​
First, we will download the SDK:
# Ensure you have Python 3.10+ installed and your virtual environment is activated
pip install truffle-sdk
Initialize Your Project Structure​
Great! Let's create our app. We will begin by going into our CLI in our IDE of choice, and run the command:
truffle init
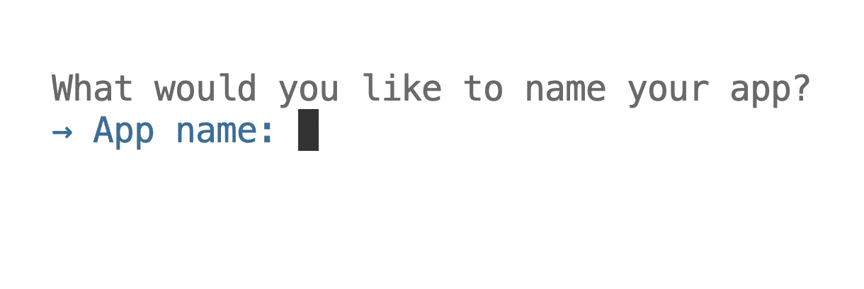
This initializes a new Truffle app. All you need to do is give a name and description of your app (and a specific path if you would like, but you do not need to specify).
The init
command creates a complete project structure with everything you need to get started.
Write Your First Tool​
We now have our project scaffolded. Let's open it up in our IDE and write some code — Remember, the magic happens inside the SDK itself, so all we need to do is write syntactically correct Python functions.
import truffle
class TruffleEcho:
def __init__(self):
self.client = truffle.TruffleClient()
@truffle.tool(
description="Echoes a Truffle salute",
icon="brain"
)
@truffle.args(prompt="Who is Truffle?")
def echo_truffle(self, prompt: str) -> str:
return "The great and mighty Truffle? All hail our overlord!"
if __name__ == "__main__":
truffle.run(TruffleEcho())
Build and Deploy​
When you upload your Truffle app, it is NOT executed on your local PC. Instead, your app is uploaded and runs on a Truffle hardware device:
- If you own a Truffle device and have it connected via Truffle OS, your app will be uploaded and run on your local Truffle hardware.
- If you do not have a device connected, your app will be uploaded and run on a Truffle hosted in the cloud.
Which Truffle is used depends on which is connected in your Truffle OS client. You can easily switch between your own device and the online Truffle in the client settings. This allows you to develop and test locally or remotely, depending on your setup.
Once our app is written, we can save and go back to the terminal. From here we will run the build command:
truffle build
The build command packages your Python function into a usable tool for your Agent. The next step is to run the upload command from the same place:
truffle upload
See Your Tool in Action​
Great! Now your app is ready to be used with your Agent. Let's go back into our Truffle Client.
You will see your newly created app here! This means your Truffle Agent is aware and will use it as needed.
Now we are back in our client.
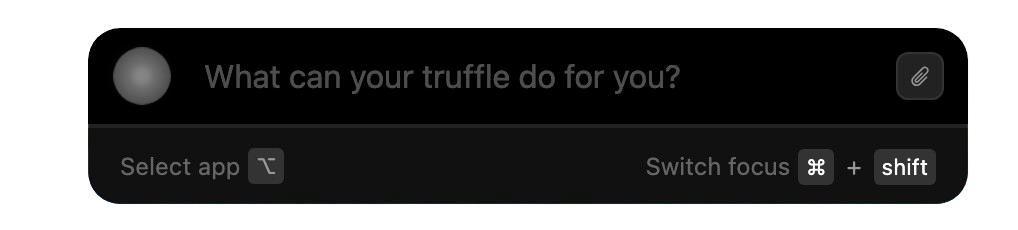
Our Agent automatically classifies as you type your prompt, and intelligently knows which tools to use best. Let's ask "What do you know about Truffle?"
Congrats, you've just created and used your first tool!
Next Steps​
Ready to build more? Check out:
- CLI Reference - Master the command line tools
- SDK Reference - Create advanced tools
- Client API - Unlock the full power of the Client API
Remember to check the Client API documentation when you're ready to add more complex interactions to your tools.